Image Sensor
This section outlines settings that are directly applied to the image sensor controls.
Acquisition Frame Rate
The acquisition needs to be stopped to adjust the frame rate. From eBus Player select...
Device Control
->Image
->interval
The parameterinterval
adjusts the inter-frame time in milliseconds. Note that the minimum arrival time is limited by resolution and practical Ethernet bandwidth considerations.
Python SDK
The interval
parameter can be obtained from the device handle in Python.
device_params = device.GetParameters()
interval = device_params.Get("interval")
res = interval.SetValue(float(<value>))
res, value = interval.GetValue()
if not res.IsOK():
# Error handling
ROS2
The ROS2 driver exposes this paramerter as fps
to set the target frames-per-second for the camera. It can be set when starting the ROS2 driver node from the commandline. Note the interval is not run-time adjustable after the driver has been started. Please see here for the required parameters to start the driver.
ros2 run bottlenose_camera_driver bottlenose_camera_driver_node --ros-args \
... \
-p fps:=<value>
Exposure and Gain Control
Exposure and gain can be adjusted while the camera is acquiring frames. From eBus Player select...
Device Control
->Image
->exposure
The parameterexposure
adjusts the exposure time of the sensor in milliseconds. Note that the maximum exposure is limited to the inter-frame time.Device Control
->Image
->gain
The parametergain
can be adjusted between unity and 22.26.Device Control
->Image
->autoExposureEnable
Enable auto exposure control. This will disable the manualexposure
andgain
controls and have the camera adjust the exposure and gain adaptively based on measured image luminance.Device Control
->Image
->dgain{Blue,GB,GR,Red}
The subcomponents of digital gain can be set on a per-colour filter pixel level.dgainBlue
Adjusts the gain for blue pixels.dgainGB
Adjusts the gain for green-blue pixels.dgainGR
Adjusts the gain for green-red pixels.dgainRed
Adjusts the gain for red pixels.pedestal
Optical black-level clamp.
Python
All of the above parameters are directly exported as part of the device handle, and can be interfaced as follows. Example for gain
shown.
device_params = device.GetParameters()
gain = device_params.Get("gain")
res = gain.SetValue(value)
res, value = gain.GetValue()
if not res.IsOK():
# Error handling
ROS2
The above parameters are exposed as exposure
, gain
, dGainBlue
, dgainGB
, dgainGR
, and dGainRed
as part of the ROS2 driver. They can be set during the start of the node and are run-time adjustable. Example for gain
shown. Please see here for the required parameters to start the driver.
ros2 run bottlenose_camera_driver bottlenose_camera_driver_node --ros-args \
... \
-p gain:=<value>
To adjust any of the values at run-time after the node has been started, you can use ros2 param {set, get}
as follows.
ros2 param get <node_name> <parameter_name>
To enable auto exposure, please use the following startup parameter. Please node enabling auto exposure will override any manual exposure and gain inputs.
ros2 run bottlenose_camera_driver bottlenose_camera_driver_node --ros-args \
... \
-p autoExposureEnable:=true
Adjusting Frame Resolution
Bottlenose supports discrete image resolutions. They can be configured when the camera is not acquiring images. To select the desired discrete resolution from the table shown below, adjust theHeight
in Device Control
in eBus Player. Note adjusting the Height
variable will automatically update the Width
variable with possible configuration shown in the table below. Other combinations will raise an error.
Width | Height | Binning | Subsampling |
---|---|---|---|
3840 | 2160 | 1:1 | 1:1 |
1920 | 1440 | 2:2 | 1:1 |
1920 | 1080 | 2:2 | 1:1 |
Python
The parameter Height
is exposed as part of the device parametes and can be set as follows.
device_params = device.GetParameters()
height = device_params.Get("Height")
res = height.SetValue(value)
res, value = height.GetValue()
if not res.IsOK():
# Error handling
ROS2
The resolution is exposed as format
parameter in ROS2. It can be set during driver start-up as follows for valid combinations.
ros2 run bottlenose_camera_driver bottlenose_camera_driver_node --ros-args \
... \
-p format:=1920x1080
Offset Control For Stereo Alignment
Stereo calibration provides the extrinsic parameters required for rectification. Rectification performs image manipulation that allows each row of the left image to be in line with each row in the right image.
Adjusting the Y-axis offset will move the images up and down in the background 12MP canvas of the image sensor.
Adjusting the Y-axis offset is mandatory for stereo alignment for Sparse Stereo and Dense Stereo functionality. Please see Stereo Calibration for best practices to set the Y-axis offset.
Adjusting the X-axis offset will move the images left and right in the background 12MP canvas. This will have the effect of tilting the optical axis inwards or outwards. An inward tilt will allow closer 3D depth measurement capability.
The offsets for the left camera are exposed as OffsetX
, OffsetY
under ImageFormatControl
in eBus Player. For Bottlenose Stereo the offsets of the right camera are exposed as OffsetX1
, OffsetY1
. The offsets are applied in the respective readout direction of the sensor as shown below. The offsets have to be divisible by two and will be applied for raw pixels, irrespective of binning.
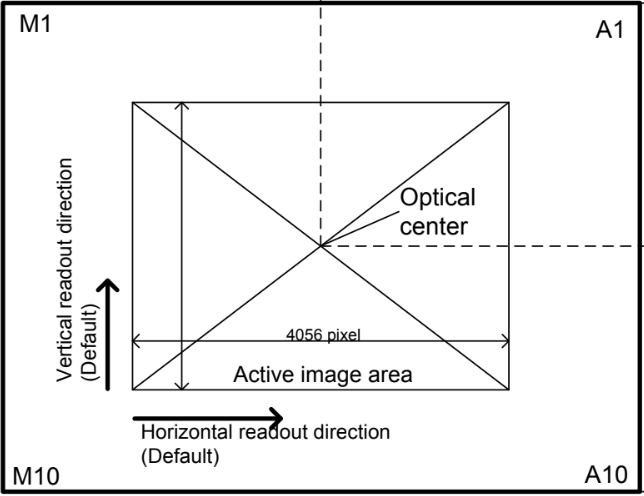
Readout Direction for Offset Control
Default offsets are shown below. They are adjusted to center the image in the optical center of the image sensor. Lens choices or mechanical tolerances can result in slightly offset center points of the left and right image sensors in Bottlenose Stereo.
With | Height | OffsetX{1,2} | OffsetY{1,2} |
---|---|---|---|
1920 | 1440 | 108 | 80 |
3840, 1920 (binned) | 2160, 1080 (binned) | 108 | 440 |
Python
The above parameters are exposed as part of the device
handle, and can be adjusted as follows. The example shown for OffsetY1
.
device_params = device.GetParameters()
offset = device_params.Get("OffsetY1")
res = offset.SetValue(value)
res, value = offset.GetValue()
if not res.IsOK():
# Error handling
ROS2
In ROS2 these parameters are exposed with the same names. Note they have to be set when the driver node starts and are not runtime adjustable. Example shown for OffsetY1
.
ros2 run bottlenose_camera_driver bottlenose_camera_driver_node --ros-args \
... \
-p OffsetY1:=440
Updated over 1 year ago